Step (1)Read the picture and find the size
>> cd c:\Users\admin\pictures
>> A=imread('dog.jpg');
>> image(A),axis off
size(A)
ans =
340 340 3
Step(2)
Shrink it down to a 34 by 34 image(dividing the original image by 10)
Octave code as follows:
function Z=zoom2(limg,scale)
rows=floor(size(limg,1)*scale);
cols=floor(size(limg,2)*scale);
for i=1:rows;
for j=1:cols;
a=round(i/scale);

b=round(j/scale);
Z(i,j,:)=limg(a,b,:);

end;
end;
endfunction
cd c:\users\admin\pictures
B=imread("dog.jpg");
B=double(B)/255;
B=zoom2(B,1/10);
imwrite("1/10dog.jpg",B(:,:,1),B(:,:,2),B(:,:,3));
size(B);
34 34 3
Step(3)Reduce the image in step 2 to 27 colors
The octave commands are as follows:

function B=draw27(A)
A=double(A);
for i=1:size(A,1);
for j=1:size(A,2);
for k=1:3
B(i,j,k)=floor(A(i,j,k)/86)*86+42;
endfor;
endfor;
endfor;
endfunction
cd c:\users\admin\pictures
A=imread("reduceddog.jpg");
B=draw27(A);
C=double(B)/255;
imwrite("dog27.jpg",C(:,:,1),C(:,:,2),C(:,:,3));
Step(4)function Z=stretch2(simg,scale)
original_rows=size(simg,1);
original_cols=size(simg,2);
rows=floor(size(simg,1)*scale);
cols=floor(size(simg,2)*scale);
for i=0:(rows-1);
for j=0:(cols-1);
a=floor((i/scale)+1);
b=floor((j/scale)+1);
if (a>0) & (a<=original_rows) & (b>0) & (b<=original_cols) Z(i+1,j+1,:)= simg(a,b,:); end; end; end; endfunction cd c:\Users\admin\pictures A=imread("dog27.jpg"); B=stretch2(A,10); size(B) 340 340 3 C=double(B)/255; imwrite("strecheddog.jpg",C(:,:,1),C(:,:,2),C(:,:,3))
Starting over: First reducing the original image into 300 x 300 size The new picture is like this:
---------------------------------------------------------------------------------------
Step(1)
cd c:\Users\admin\pictures
imread("newdog.jpg");
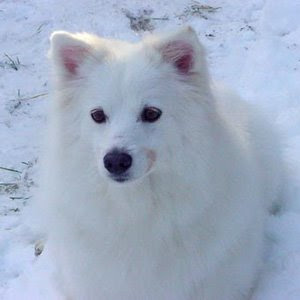
Step(2)
function Z=zoom2(limg,scale)
rows=floor(size(limg,1)*scale);
cols=floor(size(limg,2)*scale);
for i=1:rows;
for j=1:cols;
a=round(i/scale);
b=round(j/scale);
Z(i,j,:)=limg(a,b,:);
end;
end;
endfunction

octave-3.0.1.exe:10> cd c:\users\admin\pictures
octave-3.0.1.exe:11> B=imread("newdog.jpg");
octave-3.0.1.exe:12> B=double(B)/255;
octave-3.0.1.exe:13> B=zoom2(B,1/10);
octave-3.0.1.exe:14> imwrite("1/reducednewdog.jpg",B(:,:,1),B(
octave-3.0.1.exe:15> size(B);
octave-3.0.1.exe:16> size(B)
ans =
30 30 3
Step (3)
27 color reduction of the above 30 x 30 image
function B=draw27(A)
A=double(A);
for i=1:size(A,1);
for j=1:size(A,2);
for k=1:3
B(i,j,k)=floor(A(i,j,k)/86)*86+42;
endfor;
endfor;
endfor;
endfunction
cd c:\users\admin\pictures
A=imread("reducednewdog.jpg");
B=draw27(A);
C=double(B)/255;
imwrite("newdog27.jpg",C(:,:,1),C(:,:,2),C(:,:,3));
Step(4)Stretched the image in step (3) 10 times:
function Z=stretch2(simg,scale)
original_rows=size(simg,1);
original_cols=size(simg,2);
rows=floor(size(simg,1)*scale);
cols=floor(size(simg,2)*scale);
for i=0:(rows-1);
for j=0:(cols-1);
a=floor((i/scale)+1);
b=floor((j/scale)+1);
if (a>0) & (a<=original_rows) & (b>0) & (b<=original_cols) Z(i+1,j+1,:)= simg(a,b,:); end; end; end; endfunction cd c:\Users\admin\pictures A=imread("newdog27.jpg"); B=stretch2(A,10); size(B) 300 300 3 C=double(B)/255; imwrite("strechednewdog.jpg",C(:,:,1),C(:,:,2),C(:,:,3))
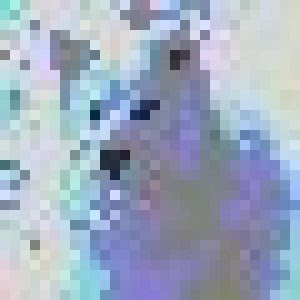
Pictures and their average colors
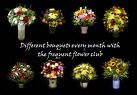
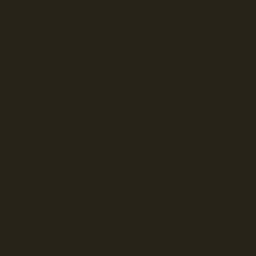





My original picture is 300x300 and now after shrinking to 30x30, changing to 27 colours, and then enlarging it back to 300x300, there are 30 square "pixels" along the top of the 300x300 picture. Therefore each 'square' is 10x10. So, I will need to resize all my 'small average colour pictures' to a 10x10 size.
If you want to put the rose over the dogs 'right eye', you will need to enter the following code.
# Put pixels into octave...
a=imread("strechednewdog.jpg");
r=imread("pixelrose.png");
# You will need to 'imread' each 10x10 picture you want to use in your mosaic above...
# Now, let's move the rose icon over the dog's right eye...
# Note that the coordinates are (y-axis, x-axis,:)...
a(110:119,90:99,:)=r
# How do you get these numbers????
# start at the dog's eye and start counting all the way to the top of the picture...you should get 11...so each pixel square is 10x10...therefore your starting coordinate for the coloumn would be 11x10=110. Your finishing coordinate for the column would be x+9...always...in this case it is 110+9=119.
# similarly, for the rows, I start at the dogs eye. Start counting to the left of the picture and you should get 9 squares. Therefore 9x10=90 is your starting coordinate for the rows and your finishing coordinate is 90+9=99...
# to see the image...
f=double(a)/255;
imwrite("dogtest.png",f(:,:,1),f(:,:,2),f(:,:,3));
Here is how the image test looks like:
cd c:\Users\admin\pictures
a=imread("newdogstretched");
ctave-3.0.1.exe:19> a=imread("newdogstretched.jpg");
ctave-3.0.1.exe:20> r2=imread("p2new.jpg");
ctave-3.0.1.exe:21> a(110:119,90:99,:)=r2;
ctave-3.0.1.exe:22> a(110:119,140:149,:)=r2;
ctave-3.0.1.exe:23> a(110:119,130:139,:)=r2;
ctave-3.0.1.exe:24> a(150:159, 100:109,:)=r2;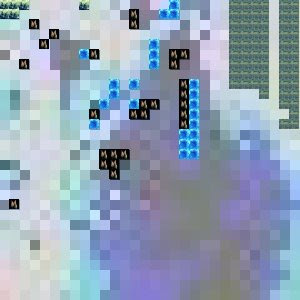
ctave-3.0.1.exe:25> a(150:159, 110:119,:)=r2;
ctave-3.0.1.exe:26> a(150:159, 120:129,:)=r2;
ctave-3.0.1.exe:27> a(100:110, 140:149,:)=r2;
ctave-3.0.1.exe:27> a(100:109, 140:149,:)=r2;
ctave-3.0.1.exe:28> a(100:109, 150:159,:)=r2;
ctave-3.0.1.exe:29> a(50:59, 0:9,:)=r2;
ctave-3.0.1.exe:29> a(50:59, 00:09,:)=r2;
ctave-3.0.1.exe:29> a(20:29,30:39,:)=r2;
ctave-3.0.1.exe:30> a(60:69,20:29,:)=r2;
ctave-3.0.1.exe:33> a(50:59,180:189,:)=r2;
ctave-3.0.1.exe:34> a(50:59,170:179,:)=r2;
ctave-3.0.1.exe:35> a(50:59, 90:99,:)=r2;
ctave-3.0.1.exe:36> a(60:69,170:179,:)=r2;
ctave-3.0.1.exe:37> a(200:209,10:19,:)=r2;
ctave-3.0.1.exe:38> a(30:39,50:59,:)=r2;
ctave-3.0.1.exe:39> a(40:49,40:49,:)=r2;
ctave-3.0.1.exe:31> f=double(a)/255;
ctave-3.0.1.exe:32> imwrite("dogtest.jpg",f(:,:,1),f(:,:,2),f(:,:,3));