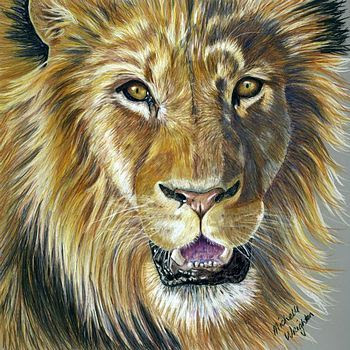
Step (1)
Picture(from Google search), saved on the disk and read with the following code.
Code in MATLAB
cd c:\users\admin\pictures
lion=imread('lionphotomosaic.jpg');
lion=double(lion)/255;
imshow(lion)
size (lion)
ans =
350 350 3
Step 2
Shrink it down to a 35 by 35 image(dividing the original image by 10)
function Z=zoom2(limg,scale)
rows=floor(size(limg,1)*scale);
cols=floor(size(limg,2)*scale);
for i=1:rows;
for j=1:cols;
a=round(i/scale);
b=round(j/scale);
Z(i,j,:)=limg(a,b,:);
end;
end;
endfunction
cd c:\users\admin\pictures
B=imread("lionphotomosaic.jpg");
B=double(B)/255;
B=zoom2(B,1/10);
imwrite("lion2mosaic.jpg",B(:,:,1),B(:,:,2),B(:,:,3));
size(B);
ans =
35 35 3

Step 3
Reduce it with only 27 color values.
Octave code is as follows( I will try to write in MATLAB too)
function B=draw27(A)
A=double(A);
for i=1:size(A,1);
for j=1:size(A,2);
for k=1:3
B(i,j,k)=floor(A(i,j,k)/86)*86+42;
endfor;
endfor;
endfor;
endfunction
cd c:\users\admin\pictures
A=imread("lion2mosaic.jpg")
lion27=draw27(A);
B=double(lion27)/255;
imwrite("lion3mosaic.jpg",B(:,:,1),B(:,:,2),B(:,:,3));

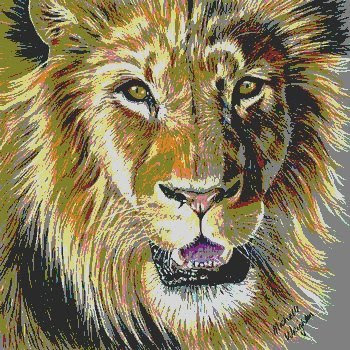
Original size(not reduced) but 27 colors
Step 4
Enlarge(10 times) the image(reduced 27 colors) using the nearest neighbor approach.
Size of the image will be 350 x 350
Octave code is like this:
function Z=stretch2(simg,scale)
original_rows=size(simg,1);
original_cols=size(simg,2);
rows=floor(size(simg,1)*scale);
cols=floor(size(simg,2)*scale);
for i=0:(rows-1);
for j=0:(cols-1);
a=floor((i/scale)+1);
b=floor((j/scale)+1);
if (a>0) & (a<=original_rows) & (b>0) & (b<=original_cols) Z(i+1,j+1,:)= simg(a,b,:); end; end; end; endfunction cd c:\Users\admin\pictures A=imread("lion3mosaic.jpg"); B=stretch2(A,10); size(B) 350 350 3 C=double(B)/255; imwrite("strechedlionlion4.jpg",C(:,:,1),C(:,:,2),C(:,:,3))
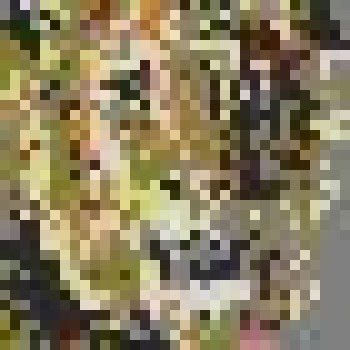
Step 5
Find at least one picture whose average color is one of the 27 possible colors that appear in the picture.
No comments:
Post a Comment